StateList vs. List<State> in Jetpack Compose: How to Optimize State Management
2024-03-08 10:37:12
StateList vs. List
Introduction
State management is crucial for building responsive and interactive user interfaces in Jetpack Compose. The choice between StateList
and List<State>
can significantly impact the performance and efficiency of your app. This article delves into the key differences between these two data structures, exploring their advantages and drawbacks to help you make informed decisions for your Compose applications.
StateList: A Direct Approach
StateList
offers a straightforward way to manage a mutable list of state objects. Modifying the list directly, such as adding or removing elements, triggers recomposition for all composables that depend on it. This simplicity makes StateList
ideal for scenarios where frequent changes to the list structure are required.
Deferring State Reading
To mitigate excessive recompositions, a technique called "deferring state reading" is recommended. Instead of accessing state directly within composables, create a new state variable and assign the desired value to it. This new variable serves as a stable reference, minimizing unnecessary recompositions.
// Example with StateList
@Composable
fun DrawNode(
node: () -> Node,
) {
val localNodeState = remember { node() }
// ...
}
List: Controlled State Management
List<State>
introduces a more controlled approach to state management. Each element in the list is represented by its own state object. Modifying individual elements' state does not trigger recomposition for the entire list, offering finer-grained control over state updates.
Deferring State Reading
Similar to StateList
, deferring state reading is also beneficial with List<State>
. By creating a new state variable and assigning it the state of the desired element, you can defer reading the original state object.
// Example with List<State>
@Composable
fun DrawNode(
nodeState: MutableState<Node>,
) {
val localNodeState = remember { nodeState.value }
// ...
}
Recomposition in StateList
Despite deferring state reading, recomposition in StateList
is unavoidable due to its mutable nature. Any change to the list structure, regardless of whether individual element states are modified, triggers recomposition. To optimize performance, it's crucial to minimize these structural changes.
Choosing the Right Data Structure
The decision between StateList
and List<State>
depends on the specific use case:
- Use StateList: When frequent modifications to the list structure are necessary.
- Use List
: When minimizing recompositions is paramount and updates are primarily focused on individual elements' state.
Conclusion
Understanding the nuances between StateList
and List<State>
empowers you to make informed choices for your Compose applications. By leveraging these data structures effectively, you can optimize performance, minimize recompositions, and deliver a seamless user experience.
Frequently Asked Questions
-
When should I use
StateList
overList<State>
?- Use
StateList
when the list structure is likely to change frequently.
- Use
-
How can I avoid unnecessary recompositions with
StateList
?- Defer state reading by creating new state variables and assigning them the desired values.
-
What are the advantages of using
List<State>
?- It offers finer-grained control over state updates, minimizing recompositions.
-
When should I defer state reading?
- Defer state reading whenever possible to improve performance.
-
How can I optimize performance with
StateList
?- Minimize structural changes to the list by updating individual elements' state instead.
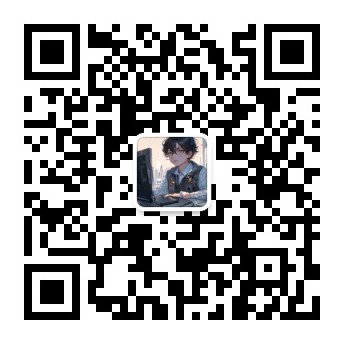
在Jetpack Compose中以优雅的方式实现数据持久化
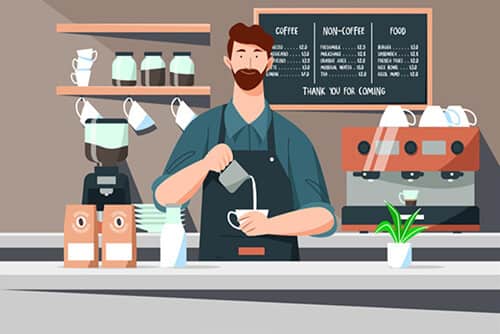
: Text('阅读器'), ), body: PageView( controller: _pageController, onPageChanged: (page) { setState(() { _currentPage = page; }); }, children: [ // 加载小说的页面 ], ), ); } } ``` ### 结论 利用 Flutter 的功能和灵活性,开发一款交互式小说阅读应用程序成为一种可能。通过实现图书馆管理、阅读器定制、内容发现、社交分享和离线阅读等关键功能,开发人员可以创建一款能够提供卓越用户体验的应用程序。结合 Flutter 的跨平台开发和卓越性能优势,小说爱好者可以在他们的移动设备上尽情享受沉浸式的阅读体验。 ### 附加说明: ****Flutter的小说世界:打造交互式阅读盛宴
![: Text('阅读器'),
),
body: PageView(
controller: _pageController,
onPageChanged: (page) {
setState(() {
_currentPage = page;
});
},
children: [
// 加载小说的页面
],
),
);
}
}
```
### 结论
利用 Flutter 的功能和灵活性,开发一款交互式小说阅读应用程序成为一种可能。通过实现图书馆管理、阅读器定制、内容发现、社交分享和离线阅读等关键功能,开发人员可以创建一款能够提供卓越用户体验的应用程序。结合 Flutter 的跨平台开发和卓越性能优势,小说爱好者可以在他们的移动设备上尽情享受沉浸式的阅读体验。
### 附加说明:
****Flutter的小说世界:打造交互式阅读盛宴](https://oss.bolzjb.com/blog/thumb/42.jpg)
OpenGL ES 3.0 深入探索:打造您自己的纹理渲染
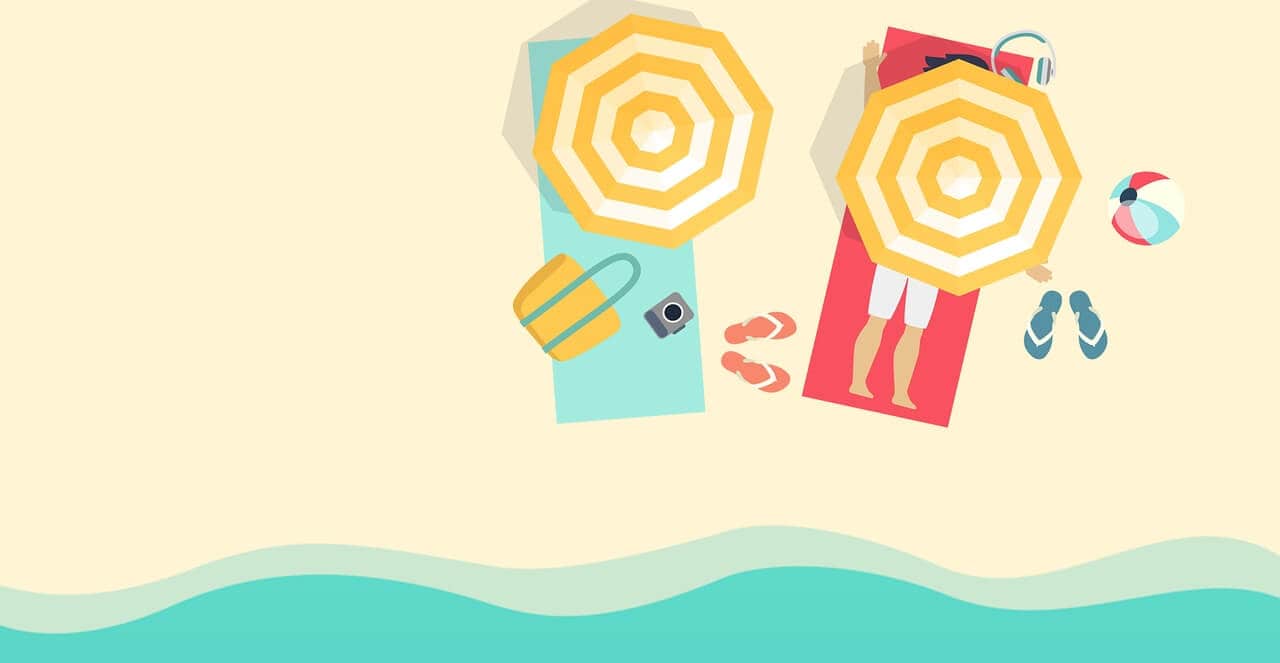
AsyncTask 代码分析:深入理解 Android 中的异步任务
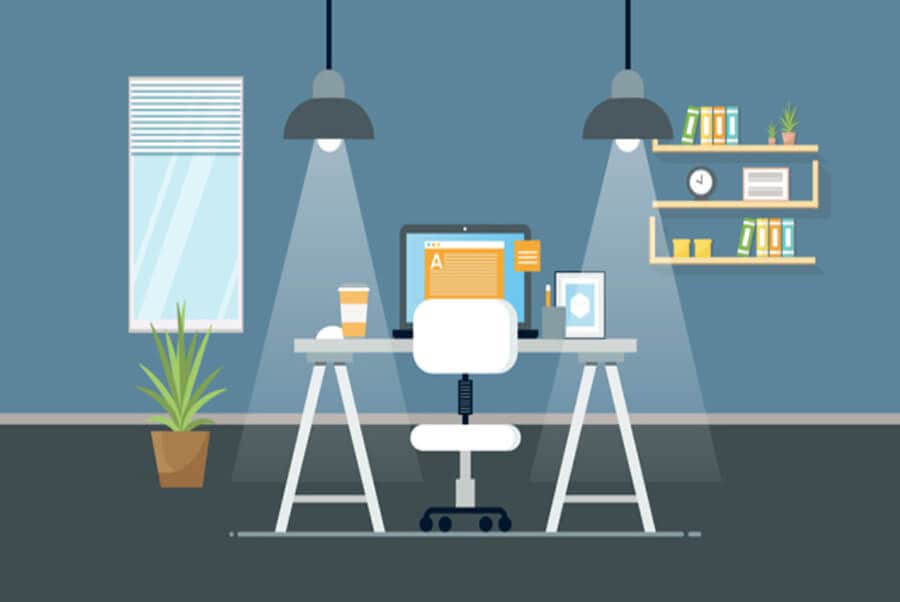
深入解读 RecyclerView 源码:探索 Recycler 的奥秘
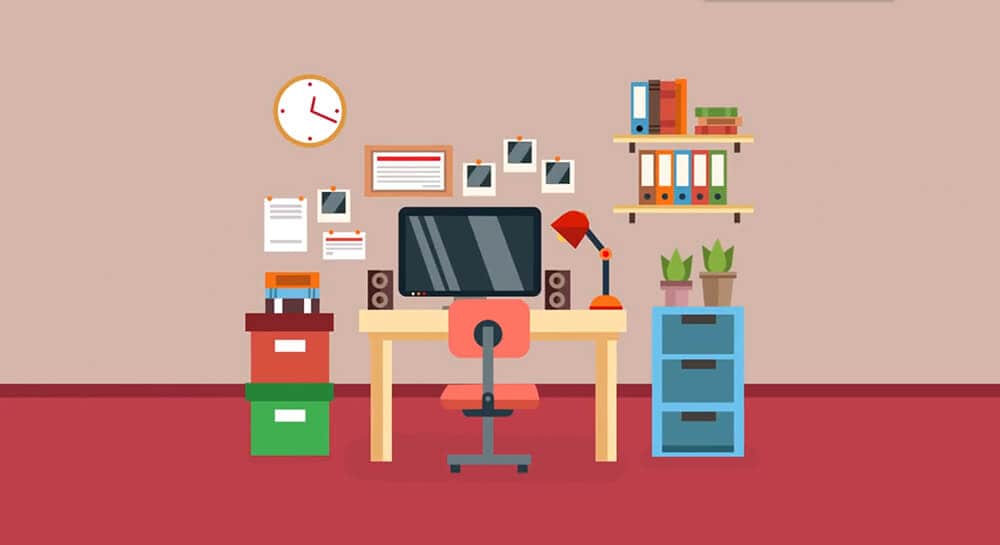