《前端面试从 ES6 开始(全面解析)》
2023-10-05 21:56:50
前言
ES6 是 JavaScript 的最新版本,于 2015 年 6 月正式发布。它引入了许多新的特性,让 JavaScript 更强大、更易用。这些新特性包括箭头函数、模板字符串、解构赋值、扩展运算符、Promise、Generator、Set、Map 和 Symbol 等。
本文将全面解析 ES6 的新特性,并提供丰富的示例代码,帮助你快速掌握 ES6 的用法。无论是前端开发新手还是经验丰富的开发人员,都能从本文中受益匪浅。
ES6 新特性
箭头函数
箭头函数是 ES6 中引入的一种新的函数定义方式。箭头函数的语法更简洁,而且可以使用更少的代码来实现相同的功能。
// ES5 函数定义方式
function sum(a, b) {
return a + b;
}
// ES6 箭头函数定义方式
const sum = (a, b) => a + b;
模板字符串
模板字符串是 ES6 中引入的一种新的字符串定义方式。模板字符串可以使用反引号(``)来定义,而且可以包含变量和表达式。
// ES5 字符串定义方式
const name = 'John Doe';
const age = 30;
const greeting = 'Hello, ' + name + '! You are ' + age + ' years old.';
// ES6 模板字符串定义方式
const name = 'John Doe';
const age = 30;
const greeting = `Hello, ${name}! You are ${age} years old.`;
解构赋值
解构赋值是 ES6 中引入的一种新的变量定义方式。解构赋值可以将数组或对象中的元素直接赋值给变量。
// ES5 数组解构赋值
const numbers = [1, 2, 3];
const first = numbers[0];
const second = numbers[1];
const third = numbers[2];
// ES6 数组解构赋值
const numbers = [1, 2, 3];
const [first, second, third] = numbers;
// ES5 对象解构赋值
const person = { name: 'John Doe', age: 30 };
const name = person.name;
const age = person.age;
// ES6 对象解构赋值
const person = { name: 'John Doe', age: 30 };
const { name, age } = person;
扩展运算符
扩展运算符是 ES6 中引入的一种新的运算符。扩展运算符可以将数组或对象中的元素展开为单个元素。
// ES5 数组扩展运算符
const numbers1 = [1, 2, 3];
const numbers2 = [4, 5, 6];
const numbers3 = numbers1.concat(numbers2);
// ES6 数组扩展运算符
const numbers1 = [1, 2, 3];
const numbers2 = [4, 5, 6];
const numbers3 = [...numbers1, ...numbers2];
// ES5 对象扩展运算符
const person1 = { name: 'John Doe', age: 30 };
const person2 = { city: 'New York', country: 'USA' };
const person3 = Object.assign({}, person1, person2);
// ES6 对象扩展运算符
const person1 = { name: 'John Doe', age: 30 };
const person2 = { city: 'New York', country: 'USA' };
const person3 = { ...person1, ...person2 };
Promise
Promise 是 ES6 中引入的一种新的异步编程模型。Promise 可以让你在异步操作完成时执行回调函数。
// ES5 异步编程
function getUser(id, callback) {
setTimeout(() => {
callback({ name: 'John Doe', age: 30 });
}, 1000);
}
getUser(1, function(user) {
console.log(user.name); // John Doe
});
// ES6 Promise 异步编程
function getUser(id) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ name: 'John Doe', age: 30 });
}, 1000);
});
}
getUser(1).then(function(user) {
console.log(user.name); // John Doe
});
Generator
Generator 是 ES6 中引入的一种新的函数类型。Generator 函数可以让你暂停函数的执行,并在稍后继续执行。
// ES5 迭代器
const numbers = [1, 2, 3, 4, 5];
const iterator = numbers.values();
for (let number of iterator) {
console.log(number); // 1, 2, 3, 4, 5
}
// ES6 Generator 迭代器
function* numbersGenerator() {
yield 1;
yield 2;
yield 3;
yield 4;
yield 5;
}
const generator = numbersGenerator();
for (let number of generator) {
console.log(number); // 1, 2, 3, 4, 5
}
Set
Set 是 ES6 中引入的一种新的数据结构。Set 是一个无序集合,它可以存储任何类型的元素,并且不会重复。
// ES5 集合
const numbers = new Set([1, 2, 3, 4, 5]);
// ES6 Set 集合
const numbers = new Set([1, 2, 3, 4, 5]);
Map
Map 是 ES6 中引入的一种新的数据结构。Map 是一个键值对集合,它可以存储任何类型的键和值,并且可以快速查找和检索数据。
// ES5 键值对集合
const person = { name: 'John Doe', age: 30 };
// ES6 Map 键值对集合
const person = new Map([
['name', 'John Doe'],
['age', 30]
]);
Symbol
Symbol 是 ES6 中引入的一种新的原始数据类型。Symbol 值是唯一的,它可以用来标识对象或属性。
// ES5 对象标识符
const person = {
name: 'John Doe',
age: 30
};
const nameSymbol = Symbol('name');
const ageSymbol = Symbol('age');
person[nameSymbol] = 'John Doe';
person[ageSymbol] = 30;
// ES6 Symbol 对象标识符
const person = {
name: 'John Doe',
age: 30
};
const nameSymbol = Symbol('name');
const ageSymbol = Symbol('age');
person[nameSymbol] = 'John Doe';
person[ageSymbol] = 30;
结语
ES6 是 JavaScript 的最新版本,它引入了许多新的特性,让 JavaScript 更强大、更易用。本文全面解析了 ES6 的新特性,并提供了丰富的示例代码,帮助你快速掌握 ES6 的用法。无论是前端开发新手还是经验丰富的开发人员,都能从本文中受益匪浅。
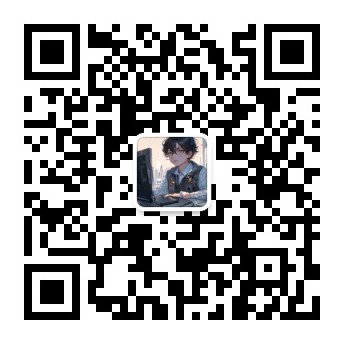
掌握Vue语法糖:简洁注册组件
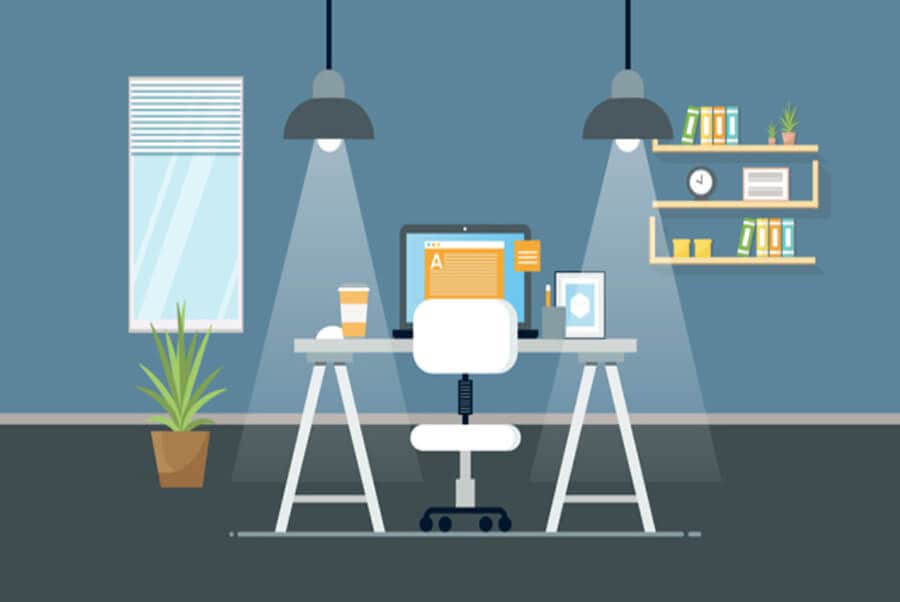
: 'Task Deadline', description: 'Complete task by 5pm' }, { date: '2023-03-15', title: 'Meeting', description: 'Meet with clients at 10am' } ] }); ``` 这将创建一个具有预定义事件的日历组件。您可以使用 Kalendar 的 API 与日历交互,添加、编辑和删除事件,或更改日历的视图。 ### 结论 Kalendar 是一个强大的日历组件,非常适合构建复杂数据的交互式日历视图。它提供了一系列特性和优势,使其成为广泛用例的理想选择。无论您是管理任务、调度资源还是可视化财务数据,Kalendar 都能提供创建交互式、高度可定制日历视图所需的工具。 日历组件 Kalendar:构建复杂数据的强大工具
![: 'Task Deadline',
description: 'Complete task by 5pm'
},
{
date: '2023-03-15',
title: 'Meeting',
description: 'Meet with clients at 10am'
}
]
});
```
这将创建一个具有预定义事件的日历组件。您可以使用 Kalendar 的 API 与日历交互,添加、编辑和删除事件,或更改日历的视图。
### 结论
Kalendar 是一个强大的日历组件,非常适合构建复杂数据的交互式日历视图。它提供了一系列特性和优势,使其成为广泛用例的理想选择。无论您是管理任务、调度资源还是可视化财务数据,Kalendar 都能提供创建交互式、高度可定制日历视图所需的工具。
日历组件 Kalendar:构建复杂数据的强大工具](https://oss.bolzjb.com/blog/thumb/41.jpg)
打破学习迷思:揭秘学习之外的至关要义
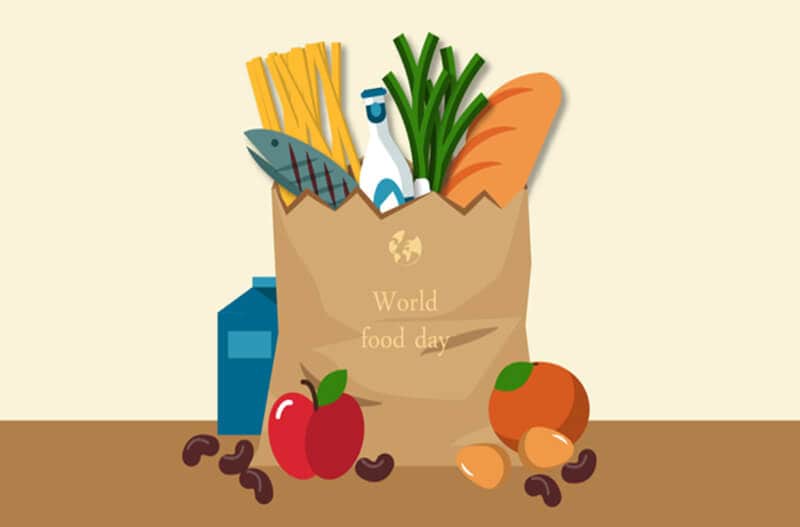
往事不堪回首:一场由 Fetch 引发的考古探索
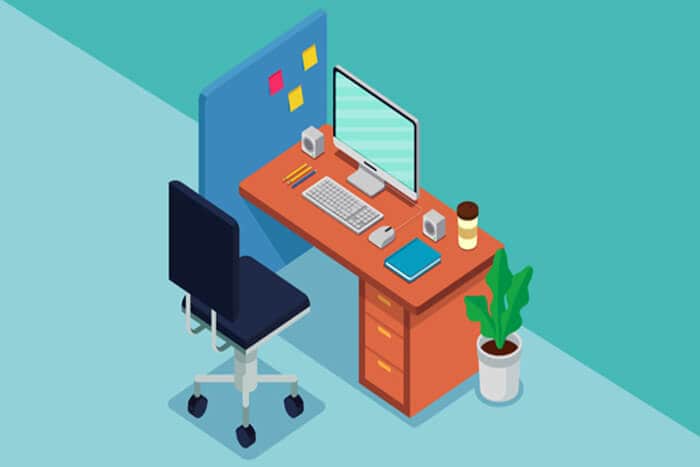
技术指南:使用 React 18 自动批处理减少渲染次数
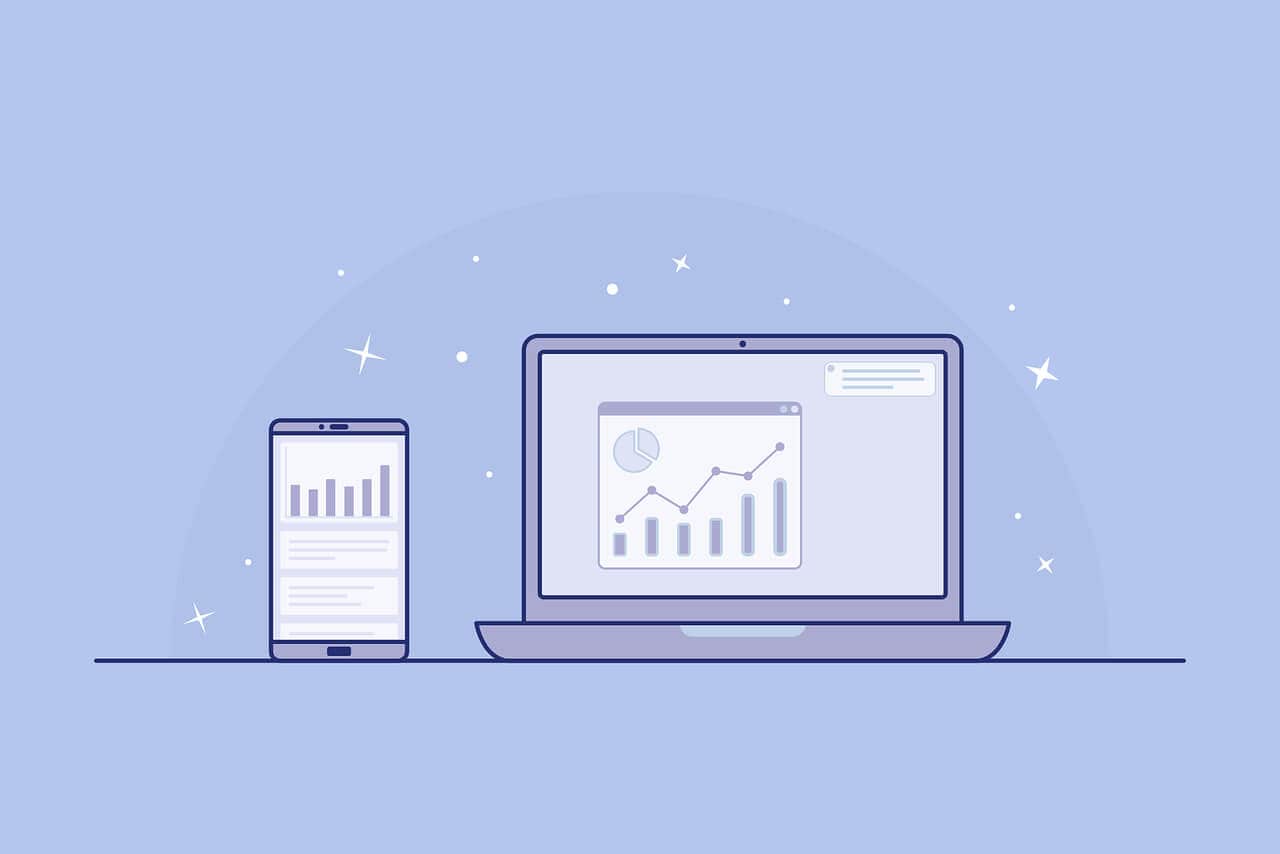