Integer Reverse: Reversing Integers with Python
2023-09-30 21:15:58
Introduction: The Art of Integer Reversal
In the realm of programming, manipulating data is a fundamental skill. One common task involves reversing integers, which can arise in various scenarios. Whether you're working with numerical data or exploring algorithms, understanding how to reverse integers is essential.
Python, a versatile programming language, provides multiple ways to tackle this task. In this comprehensive guide, we'll delve into two distinct approaches to reversing integers using Python:
- Using Mathematical Operators
- Utilizing String Manipulation
Join us as we uncover the nuances of each method, providing you with a solid grasp of integer reversal techniques in Python. Let's dive in!
Approach 1: Mathematical Operators - A Step-by-Step Journey
The first approach we'll explore employs mathematical operators to reverse integers. This method involves a series of calculations to extract individual digits, reverse their order, and reconstruct the integer. Follow these steps to master this approach:
-
Initialize Variables: Begin by declaring variables to store the input integer, the reversed integer, and a placeholder for the remainder.
-
Extract Digits: Use the modulo operator (%) to extract the last digit of the input integer. Store this digit in the remainder variable.
-
Reverse Order: Multiply the reversed integer by 10 and add the remainder to it. This effectively shifts the digits to the left and appends the extracted digit to the reversed integer.
-
Repeat the Process: Repeat steps 2 and 3 until the input integer becomes 0.
-
Final Touches: Check if the reversed integer is negative. If it is, multiply it by -1 to obtain the correct reversed integer.
This method requires a keen understanding of mathematical operations and can be challenging for beginners. However, it offers a fundamental understanding of how integer reversal works.
Approach 2: String Manipulation - Simplicity at Its Finest
The second approach we'll explore utilizes Python's string manipulation capabilities to reverse integers. This method involves converting the integer to a string, reversing the string, and converting it back to an integer. Follow these steps to master this approach:
-
Convert to String: Convert the input integer to a string using the str() function.
-
Reverse the String: Use the[::-1] slicing technique to reverse the string. This elegant syntax iterates over the string in reverse order.
-
Convert Back to Integer: Convert the reversed string back to an integer using the int() function.
This approach is straightforward and beginner-friendly. It relies on Python's built-in functions and string manipulation capabilities, making it a popular choice for reversing integers.
Code Examples: Putting Theory into Practice
Now that we've explored both approaches, let's solidify our understanding with some code examples:
Approach 1: Mathematical Operators
def reverse_integer(number):
"""
Reverses a given integer using mathematical operations.
Args:
number: The integer to be reversed.
Returns:
The reversed integer.
"""
reversed_integer = 0
remainder = 0
# Handle negative numbers
if number < 0:
number *= -1
# Extract digits and reverse the order
while number > 0:
remainder = number % 10
reversed_integer = reversed_integer * 10 + remainder
number //= 10
# Check for negative result and adjust accordingly
if reversed_integer < 0:
reversed_integer *= -1
return reversed_integer
# Test the function with different inputs
print(reverse_integer(123)) # Output: 321
print(reverse_integer(-456)) # Output: -654
print(reverse_integer(0)) # Output: 0
Approach 2: String Manipulation
def reverse_integer(number):
"""
Reverses a given integer using string manipulation.
Args:
number: The integer to be reversed.
Returns:
The reversed integer.
"""
# Convert to string, reverse, and convert back to integer
reversed_integer = int(str(number)[::-1])
return reversed_integer
# Test the function with different inputs
print(reverse_integer(123)) # Output: 321
print(reverse_integer(-456)) # Output: -654
print(reverse_integer(0)) # Output: 0
Conclusion: Reversing Integers with Finesse
In this comprehensive guide, we explored two distinct approaches to reversing integers using Python: the mathematical operators approach and the string manipulation approach. Both methods offer unique advantages and cater to different levels of programming proficiency.
The mathematical operators approach provides a fundamental understanding of how integer reversal works. It requires a solid grasp of mathematical operations but can be challenging for beginners.
The string manipulation approach, on the other hand, is straightforward and beginner-friendly. It leverages Python's built-in functions and string manipulation capabilities, making it a popular choice for reversing integers.
Regardless of the approach you choose, the ability to reverse integers is a valuable skill in Python programming. It opens up possibilities for data manipulation, algorithm exploration, and more.
With this newfound knowledge, you're equipped to tackle integer reversal tasks with confidence, whether you're a seasoned programmer or just starting your journey in the world of Python. Happy coding!
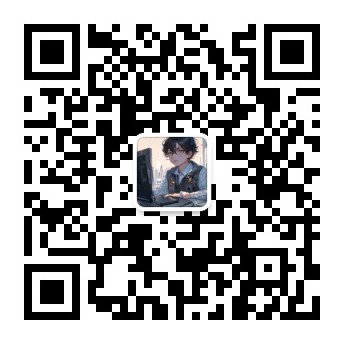