返回
重建二叉树的奥秘:从前序和中序遍历中探寻结构
前端
2023-12-03 01:56:37
导言
在计算机科学的世界里,二叉树是一种无处不在的数据结构,它以其高效性和灵活性而闻名。二叉树的重建是一个基本而有趣的问题,它要求我们从给定的序列中恢复树的原始结构。
问题陈述
给定一棵二叉树的前序遍历序列 {1, 2, 4, 7, 3, 5, 6, 8} 和中序遍历序列 {4, 7, 2, 1, 5, 3, 8, 6},重建这棵二叉树。
解决方案
要重建二叉树,我们可以利用前序和中序遍历之间的关系。前序遍历序列的第一个元素是根节点,而中序遍历序列中的左右子树将该根节点分隔开来。
算法
def reconstruct_tree(preorder, inorder):
"""重建一棵二叉树。
参数:
preorder: 前序遍历序列。
inorder: 中序遍历序列。
返回:
根节点。
"""
if not preorder or not inorder:
return None
# 根节点是前序遍历序列的第一个元素。
root_val = preorder[0]
# 找到中序遍历序列中根节点的位置。
root_index = inorder.index(root_val)
# 左子树的前序和中序遍历序列。
left_preorder = preorder[1:root_index + 1]
left_inorder = inorder[:root_index]
# 右子树的前序和中序遍历序列。
right_preorder = preorder[root_index + 1:]
right_inorder = inorder[root_index + 1:]
# 递归重建左子树和右子树。
left_child = reconstruct_tree(left_preorder, left_inorder)
right_child = reconstruct_tree(right_preorder, right_inorder)
# 创建根节点并返回。
root = TreeNode(root_val)
root.left = left_child
root.right = right_child
return root
示例
使用给定的前序遍历序列 {1, 2, 4, 7, 3, 5, 6, 8} 和中序遍历序列 {4, 7, 2, 1, 5, 3, 8, 6},我们可以重建如下二叉树:
1
/ \
2 3
/ \ \
4 5 6
/ \
7 8
总结
通过利用前序和中序遍历序列中隐含的关系,我们可以有效地重建二叉树。这一算法在计算机科学中有着广泛的应用,从数据结构的表示到语法分析。掌握这一技巧将为我们解决更复杂的数据结构问题奠定基础。
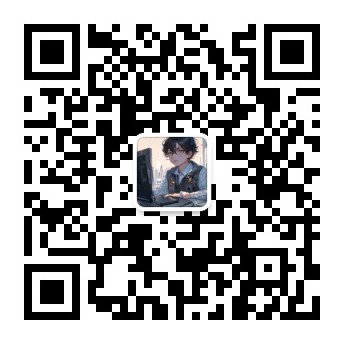
扫码关注微信公众号